Unity (C#)
Game Development
Space Escape
I have created a side-scroller game on unity called “Space Escape”. The mission of the game is to save planet earth from incoming aliens. Below is a list of controls to play the game.
Space Bar —> Shoot
Up Arrow —> Move Up
Down Arrow —> Move Down
Left Arrow —> Move Left
Right Arrow —> Move Right
The enemy functions with two scripts called EnemyController and EnemyShoot.
EnemyController: Makes the enemy starts and resets at a random y. The axis between -4.5 and 4.5. Also when the enemy collides with the players’ bullet it will give the player 2 points, shows explosion animation and sound and resets.
EnemyShoot: Allows the enemy to shoot once the distance between the player and enemy is less than 10 and the shooting has a time delay of 0.2f.
Score and health instances are created in the “Player” script.
- When the player collides with an enemy, the player loses 10 health (in PlayerCollider).
- When the player collides with an enemy bullet, the player loses 5 health (in PlayerCollider).
- When Enemy collides with the players’ bullet, the enemy is reset and the player gains 2 points (in “EnemyController”).
JAVA
Pendulum Project
The following program is a swinging pendulum written in java.
- The horizontal position of the pendulum will follow the mouse, and Animation starts when you release the mouse button.
- This program also allows you to change gravity g (Click near the tip of the red arrow, and drag the mouse button to change it (up-down).
- You can also change the mass of the bob (Click near the bottom of the black stick, And drag the mouse button to change it (up-down).
Quiz
Using Java, I have developed an application for a Quiz. The application creates a Quiz and displays a Progress Report. The user enters his/her last & first name at the beginning of the quiz. The quiz contains four multiple choice questions. The user is given two attempts to answer each question while The application keeps track of the number of attempts for each question.
For any question, the user can score:
First attempt 25 marks
Second attempt 15 marks
If the user responds to a question incorrectly, the program displays the correct answer with an appropriate message and Indicates that the mark will be 0.
When the user ends the quiz, the application displays a tabular Progress Report (on
the screen itself), indicating the following:
- Current date and time
- Full Name of the user
- Number of attempts for each question
- Marks scored in each attempt
- Marks scored for the quiz
- Percentage score
AUTOCOMPLETE (Group of 3)
Autocomplete is an important feature of many modern applications. As the user types, the program predicts the complete query (typically a word phrase) that the user intends to type.
In this assignment, I have implemented autocomplete by sorting the queries in lexicographic order; using binary-search to find the set of queries that start with a given prefix and sorting the matching queries in descending order by weight. This was done using the Eclipse IDE.
I have been provided two files from my instructor to complete this task.
- The file wikitionary.txt contains the 10,000 common words in Project Gutenberg, with weights equal to their frequencies.
- The file cities.txt contains over 90,000 cities, with their weights equal to their population
WEB (.NET, C#, SQL..)
Web Development
Sports Pro (Web Application with SQL database)
This web application is called SportPro and is used by the technical support department of a hypothetical software company that develops software for sports leagues. The purpose of the application is to track technical support service calls (referred to as incidents) in a database that also stores information about the company's customers, software products, and technicians.
The application uses a database called TechSupport. The completed application will provide the following features:
- Display customers
- Create a contact list
- Survey customers
- Maintain products
- Maintain customers
It also has a separate maintenance section to allow administrator users to maintain customers and products tables. This functionality was implemented by using ASP.NET Data Source Controls (SqlDataSource) and respective Data-Bound Controls (GridVIew, and DetailsView).
The TechSupport database consists of the six tables shown in the diagram that follows. The main table is the Incidents table, which contains one row for each technical support incident.
Each row in the Incidents table is related to one row in each of the tables.
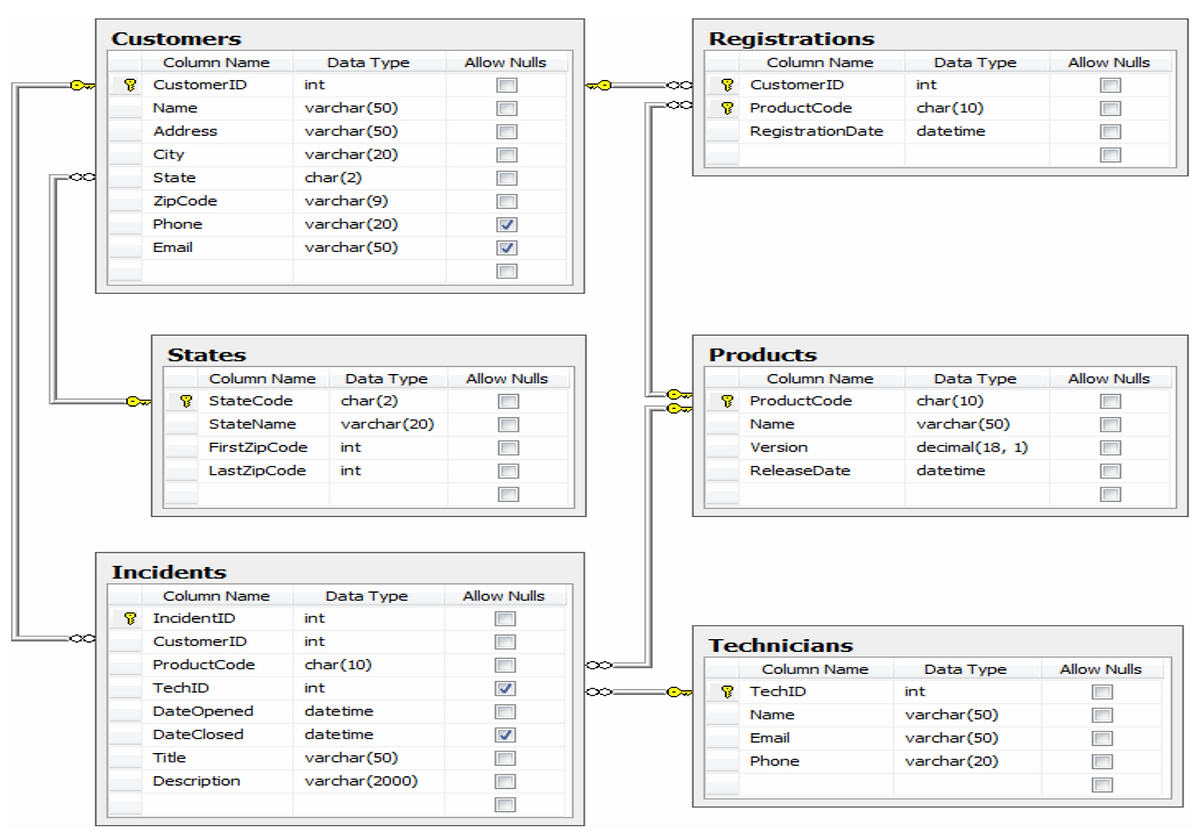